From Vue 2 dashboard to Remix: a modernization journey
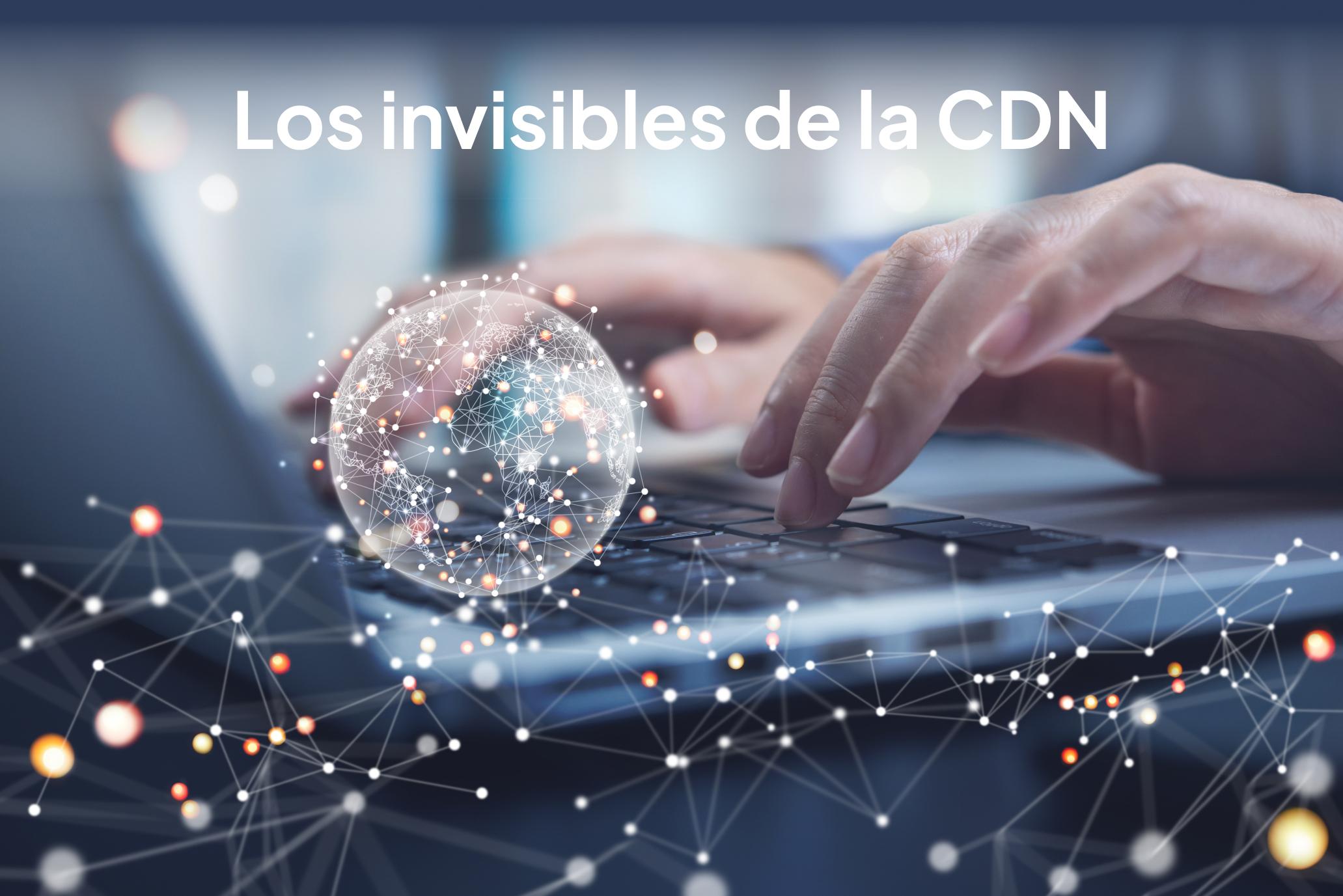
29 Jan 25
Migrating a web application can be a daunting task, especially when dealing with complex dashboards. However, the benefits of modern frameworks like Remix often outweigh the initial effort.
This post outlines the key considerations and steps involved in migrating a Vue 2 dashboard application to Remix, with a focus on handling client-side and server-side actions and data loading.
Remix offers several advantages for building modern web applications:
A step-by-step approach is recommended for migrating a Vue 2 dashboard to Remix:
Understanding the distinction between client-side and server-side operations is crucial in Remix:
Loaders: these functions run on the server and are responsible for fetching data. They are called:
Actions: these functions also run on the server and handle data mutations, such as form submissions. They are called when:
method="post"
, method="put"
, or method="delete"
is clickedThis separation of concerns simplifies data management and improves performance.
Consider a Vue 2 component that displays a table of data fetched from an API:
<template>
<table>
<tr v-for="item in items" :key="item.id">
<td>{{ item.name }}</td>
<td>{{ item.value }}</td>
</tr>
</table>
</template>
<script>
export default {
data() {
return {
items: [],
};
},
mounted() {
fetch('/api/data')
.then(res => res.json())
.then(data => {
this.items = data;
});
},
};
</script>
In Remix, this would be implemented using a loader:
import { useLoaderData } from '@remix-run/react';
export async function loader() {
const res = await fetch('/api/data');
const data = await res.json();
return data;
}
export default function DataTable() {
const items = useLoaderData();
return (
<table>
{items.map(item => (
<tr key={item.id}>
<td>{item.name}</td>
<td>{item.value}</td>
</tr>
))}
</table>
);
}
Key changes:
useLoaderData
hook provides the data to the component.Migrating from Vue 2 to Remix requires careful planning and execution. However, the benefits of Remix, such as improved performance, SEO, and developer experience, make it a worthwhile investment. By understanding the concepts of loaders and actions, and by following a step-by-step migration strategy, you can successfully modernize your dashboard application.